How to use docker run
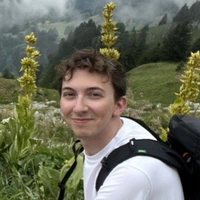
Let's talk about the docker run
command, a fundamental tool for creating and running Docker containers. This command is straightforward but packed with options that let you tailor your container's environment to suit your needs.
Creating and Running a Container
The basic syntax for docker run
is:
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
This command does a few key things:
- Creates a new container from the specified image.
- Pulls the image if it's not already on your system.
- Starts the container and runs the specified command inside it.
For instance, if you want to run a simple Ubuntu container that prints "Hello, Docker!", you could use:
docker run ubuntu echo "Hello, Docker!"
This command will pull the Ubuntu image if it's not already available, create a new container from it, and then run the echo
command inside the container.
Naming Your Container
You can give your container a custom name using the --name
flag. This makes it easier to manage and reference your containers later. For example:
docker run --name my-ubuntu-container ubuntu
This starts an Ubuntu container named my-ubuntu-container
. You can then use this name to interact with the container, like stopping it:
docker stop my-ubuntu-container
Running in Detached Mode
If you want your container to run in the background, use the -d
or --detach
flag. This is useful for long-running services. For example, to start an Nginx server in the background:
docker run -d --name my-nginx nginx
This command starts an Nginx container in detached mode, allowing you to continue using your terminal for other tasks.
Allocating Resources
You can control the resources your container uses. For instance, to limit the memory available to a container, use the -m
or --memory
flag:
docker run -m 512m ubuntu
This limits the container's memory usage to 512 megabytes.
Setting Environment Variables
You can set environment variables inside the container using the -e
or --env
flag. This is handy for configuring applications without modifying the image. For example:
docker run -e MY_VAR=hello ubuntu echo $MY_VAR
This sets an environment variable MY_VAR
to hello
and then echoes it inside the container.
Exposing and Publishing Ports
To make a container's ports accessible from the host, use the -p
or --publish
flag. For example, to expose port 80 of an Nginx container to port 8080 on the host:
docker run -p 8080:80 nginx
This maps port 8080 on the host to port 80 inside the container.
Mounting Volumes
You can mount directories from your host into the container using the -v
or --volume
flag. This is useful for persisting data. For instance:
docker run -v /host/path:/container/path ubuntu
This mounts the /host/path
directory on your host to /container/path
inside the container.
Cleaning Up
If you want Docker to automatically remove the container and its anonymous volumes after it exits, use the --rm
flag:
docker run --rm ubuntu echo "Hello, Docker!"
This command runs the container, prints the message, and then cleans up after itself.
Restart Policies
You can set a restart policy for your container using the --restart
flag. For example, to ensure a container always restarts unless it's manually stopped:
docker run --restart unless-stopped nginx
This policy tells Docker to restart the Nginx container unless it's explicitly stopped.
Interactive Mode
For interactive sessions, use the -i
or --interactive
flag along with the -t
or --tty
flag to allocate a pseudo-TTY:
docker run -it ubuntu bash
This starts an Ubuntu container and opens a bash shell inside it, allowing you to interact with the container directly.
Additional Options
There are many more options available with docker run
, such as:
--add-host
to add custom host-to-IP mappings.--cap-add
and--cap-drop
to add or remove Linux capabilities.--device
to add host devices to the container.--network
to connect the container to a specific network.
Each of these options allows you to fine-tune your container's environment and behavior, making docker run
a versatile tool for managing containers.