How to Run PostgreSQL in Docker: A Beginner's Guide
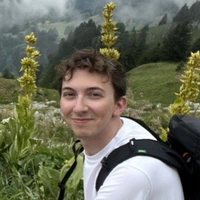
Docker lets you run PostgreSQL without installing it directly on your computer. This means you can start a database in seconds, delete it when you're done, and never worry about conflicts with other software.
This guide walks you through setting up PostgreSQL in a container, making sure your data stays safe, and following good practices when using databases in Docker.
Prerequisites
Before starting, you need:
- Docker installed on your computer. You can download it from Docker's website.
- Basic knowledge of using the command line to type commands.
Running PostgreSQL in Docker (Quick Start)
To start PostgreSQL in a container, open your terminal and type:
docker run --name postgres-container -e POSTGRES_USER=myuser -e POSTGRES_PASSWORD=mypassword -e POSTGRES_DB=mydb -p 5432:5432 -d postgres:latest
Let's break down what each part does:
--name postgres-container
: Gives your container a name so you can find it easily later-e POSTGRES_USER=myuser
: Creates a database user called "myuser"-e POSTGRES_PASSWORD=mypassword
: Sets the password for that user-e POSTGRES_DB=mydb
: Creates a database called "mydb"-p 5432:5432
: Makes PostgreSQL available on port 5432 on your computer-d postgres:latest
: Uses the newest PostgreSQL image and runs it in the background
To check if your container is running, type:
docker ps
You should see your postgres-container in the list, which means it's working.
Connecting to the PostgreSQL Container
Using Docker Exec (Command Line)
To connect directly to your database from the command line:
docker exec -it postgres-container psql -U myuser -d mydb
This opens PostgreSQL's command tool inside the container. Try typing this to check that everything works:
SELECT version();
You should see the PostgreSQL version information. To exit, type \q
and press Enter.
Using psql from Your Computer
If you already have PostgreSQL tools installed on your computer, you can connect to the container:
psql -h localhost -p 5432 -U myuser -d mydb
When asked, type the password you set earlier (mypassword
).
Connecting with a Database Tool
You can also use programs like DBeaver, pgAdmin, or TablePlus to connect to your database. Use these settings:
- Host:
localhost
- Port:
5432
- User:
myuser
- Password:
mypassword
- Database:
mydb
Persistent Storage: Keeping Data After Restart
By default, if you stop or remove your Docker container, all the data inside the database disappears. To fix this, we need to use a Docker volume to store data outside the container.
docker run --name postgres-container -e POSTGRES_USER=myuser -e POSTGRES_PASSWORD=mypassword -e POSTGRES_DB=mydb -p 5432:5432 -v pgdata:/var/lib/postgresql/data -d postgres:latest
The new part -v pgdata:/var/lib/postgresql/data
creates a storage space called "pgdata" that lives outside the container. Now your data will stay safe even if the container stops.
Running PostgreSQL with Docker Compose
Docker Compose helps you set up containers using a configuration file instead of long commands. This makes it easier to restart your database with the same settings.
Create a file called docker-compose.yml
with this content:
version: '3.8'
services:
postgres:
image: postgres:latest
container_name: postgres-container
restart: always
environment:
POSTGRES_USER: myuser
POSTGRES_PASSWORD: mypassword
POSTGRES_DB: mydb
ports:
- "5432:5432"
volumes:
- pgdata:/var/lib/postgresql/data
volumes:
pgdata:
Start PostgreSQL using this file by running:
docker-compose up -d
To stop it, run:
docker-compose down
This approach is much cleaner than typing the full docker run command each time.
Best Practices for Running PostgreSQL in Docker
Security
When using PostgreSQL in Docker:
- Don't allow direct access from the internet - use a firewall or networking rules
- Choose strong passwords, especially in production
- Create different database users for different applications instead of sharing one account
Performance Tuning
Docker containers have limits on memory and CPU. You can adjust PostgreSQL settings for better performance:
environment:
POSTGRES_USER: myuser
POSTGRES_PASSWORD: mypassword
POSTGRES_DB: mydb
POSTGRES_INITDB_ARGS: "--data-checksums"
POSTGRES_HOST_AUTH_METHOD: "md5"
POSTGRES_SHARED_BUFFERS: "256MB"
POSTGRES_WORK_MEM: "16MB"
These settings help PostgreSQL use resources more wisely inside the container.
Automatic Backups
Create a backup of your database with:
docker exec -t postgres-container pg_dump -U myuser mydb > backup.sql
This saves all your database content to a file called backup.sql
on your computer.
Deploying PostgreSQL the Easy Way: Using Sliplane
Running PostgreSQL locally is useful for development, but when you need to run it for real applications, you might want to use a managed service like Sliplane.
Sliplane handles the hard parts of running Docker containers like:
- Setting up storage that doesn't lose data
- Configuring the network correctly
- Keeping the database running if something crashes
- Creating automatic backups
- Making sure your database can handle the traffic it receives
With Sliplane, you can deploy the same PostgreSQL container we've been working with, but without worrying about the technical details of keeping it running properly.
Frequently Asked Questions
Can I run multiple PostgreSQL containers at the same time?
Yes, you can run several PostgreSQL containers simultaneously. Just make sure to:
- Use different container names for each one
- Map each container to different ports on your computer (e.g., 5432, 5433, 5434)
- Use separate volume names if you want separate data storage
Example for a second PostgreSQL container:
docker run --name postgres-container2 -e POSTGRES_USER=myuser2 -e POSTGRES_PASSWORD=mypassword2 -e POSTGRES_DB=mydb2 -p 5433:5432 -v pgdata2:/var/lib/postgresql/data -d postgres:latest
How do I connect PostgreSQL to other Docker containers?
If you want your application container to talk to PostgreSQL, you have two options:
- Using Docker Compose networks: Docker Compose creates a network for your containers automatically. Just reference the service name:
version: '3.8'
services:
postgres:
image: postgres:latest
# PostgreSQL settings...
myapp:
image: myapplication:latest
environment:
DATABASE_URL: "postgresql://myuser:mypassword@postgres:5432/mydb"
depends_on:
- postgres
- Using Docker networks manually: Create a network and connect containers:
docker network create mynetwork
docker run --name postgres-container --network mynetwork [other options] postgres:latest
docker run --name myapp --network mynetwork [other options] myapplication:latest
Inside the application container, you would connect to postgres-container:5432
instead of localhost:5432
.
How do I update my PostgreSQL container to a newer version?
To update PostgreSQL, follow these steps:
- Back up your data:
docker exec -t postgres-container pg_dump -U myuser mydb > backup.sql
- Stop and remove the old container:
docker stop postgres-container
docker rm postgres-container
- Pull the newer PostgreSQL image:
docker pull postgres:newer-version
- Start a new container with the same volume:
docker run --name postgres-container -e POSTGRES_USER=myuser -e POSTGRES_PASSWORD=mypassword -e POSTGRES_DB=mydb -p 5432:5432 -v pgdata:/var/lib/postgresql/data -d postgres:newer-version
The volume (pgdata
) contains your database files, so your data will be preserved. PostgreSQL handles version upgrades automatically when it starts.
What's the difference between using a volume and a bind mount?
- Volume (
-v pgdata:/var/lib/postgresql/data
): Docker manages the storage location on your disk. This is simpler and portable across operating systems. - Bind mount (
-v /path/on/your/computer:/var/lib/postgresql/data
): You specify exactly where on your computer the data is stored. This is useful when you want direct access to the database files.
For most users, volumes are recommended for simplicity and compatibility.
Wrapping Up
Now you know how to:
- Start PostgreSQL in Docker with a single command
- Keep your data safe using volumes
- Use Docker Compose for a cleaner setup
- Follow good practices for security and backups
Docker makes PostgreSQL much easier to use for development, and services like Sliplane can help when you're ready to use your database in a real application.
The best part is you can set up a new database whenever you need one, without installing anything directly on your computer. And when you're done, you can remove it completely with docker rm postgres-container
.